Have you ever wondered how to transfer data or interact with web APIs using Python? With cURL and its Python libraries, you can do that without much effort.
This article (for both beginners and advanced users) provides a complete guide for integrating cURL with Python. As you read through, you will learn to execute basic and advanced cURL commands in Python. You will also learn advanced techniques like handling JSON data, customizing HTTP headers, and managing file uploads and downloads. In the last section, we will go through the best practices for optimizing performance and ensuring secure HTTP requests.
Without further ado, let’s begin.
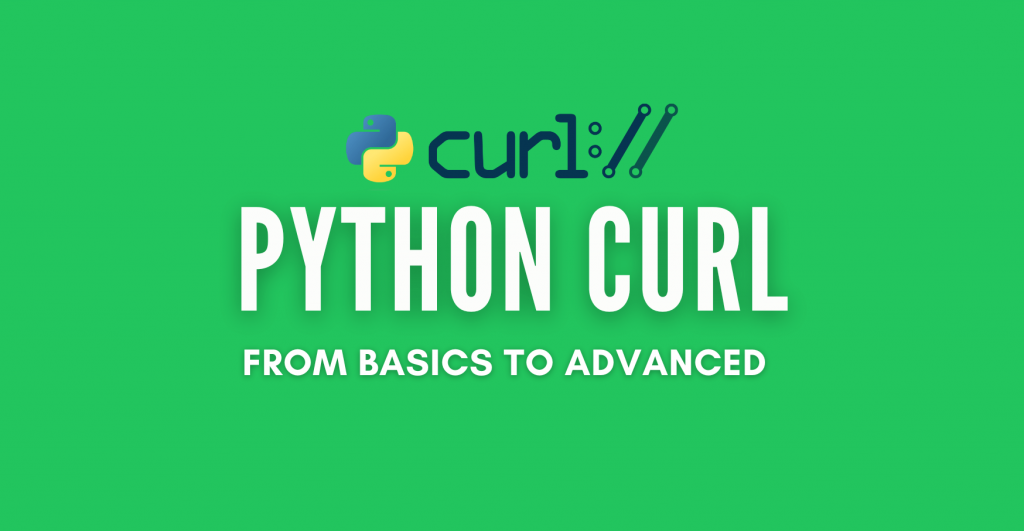
Disclaimer: This material has been developed strictly for informational purposes. It does not constitute endorsement of any activities (including illegal activities), products or services. You are solely responsible for complying with the applicable laws, including intellectual property laws, when using our services or relying on any information herein. We do not accept any liability for damage arising from the use of our services or information contained herein in any manner whatsoever, except where explicitly required by law.
Table of Contents
- Introduction to cURL
- Setting Up cURL in Python
- Setting up PycURL
- Setting up Requests
- Basic cURL Commands in Python
- Basic Commands with Requests
- Basic Commands with PycURL
- Advanced cURL Techniques
- Advanced Techniques with Requests
- Advanced Techniques with PycURL
- Best Practices, Tips, and Troubleshooting
- Security Considerations
- Optimizing Performance
- Debugging Tips
- Common Errors and Solutions
- FAQ for Python cURL
- Final Words
1. Introduction to cURL
cURL is a powerful CLI tool (and library) that lets you transfer data using URLs, with protocols like HTTP, FTP, and SFTP. It’s been around for a while, since 1998, and it’s been used in a lot of different devices and programs. cURL also comes with a lot of features, like support for TLS certificates, HTTP/2 and HTTP/3, proxy tunneling, and many authentication methods.
If you’re a coder, cURL is a must-have. You can use it in scripts, command lines, and even on embedded devices. Plus, this tool is also super helpful for web scraping, API interactions, and data processing. One way to make cURL even better is to use it with Python. There are libraries like PycURL and Requests that can give you even more features and make it easier to handle HTTP requests.
In this guide, we will focus on how to set up cURL with Python, learn a few basic cURL commands, and a few more advanced techniques.
If you’re new to this tool and you want to use cURL with proxies, we recommend you read the following beginner’s guide on using cURL with proxies. This guide will teach you everything you need to know, from installing cURL to using it with proxies. The guide also covers different proxy protocols and how to use them with cURL.
2. Setting Up cURL in Python
To set up and use cURL in Python, you can use the PycURL and Requests libraries.
PycURL is a Python interface to the cURL library. This library allows you to perform network operations like sending HTTP requests. The other library is the requests library— a powerful and user-friendly HTTP library for Python. The requests library is designed for simple HTTP request sending.
Here’s a comparison table for the Requests and PycURL libraries:
Feature | Requests | PycURL |
Ease of Use | Very easy to use. It comes with a simple and intuitive API | More complex. It requires libcurl knowledge. |
Readability | Clean and easy to read | More verbose and complex |
Performance | Good for most use cases | High performance. It is efficient for large data transfers |
Control | High-level, limited control over low-level details | Fine-grained control over request details |
Supported Protocols | HTTP/HTTPS | HTTP, HTTPS, FTP, FTPS, SCP, SFTP, and more |
Installation | pip install requests | pip install pycurl. It may need additional system dependencies |
Automatic Content Decoding | Yes | No |
SSL/TLS Verification | Yes, out-of-the-box | Requires manual setup |
Authentication | Supports various types (Basic, Digest, OAuth) | Supports various types (Basic, Digest, NTLM, etc.) |
Cookie Handling | Automatic | Manual setup required |
Proxy Support | Yes | Yes |
Best For | Beginners, general-purpose HTTP requests | Advanced users, high-performance needs, specialized protocols |
a. The guide for setting up PyCurl and Requests.
The following command will get you started with cURL in Python using the PycURL library.
Setting up PycURL:
Install PycURL:
1 |
pip install pycurl |
On Ubuntu, additional packages might be needed:
1 |
sudo apt install libcurl4-openssl-dev libssl-dev |
b. Setting up Requests.
The following command will get you started with cURL functionalities in Python using the requests libraries.
Install Requests:
1 |
pip install requests |
3. Basic cURL Commands in Python
Now that you have either library installed, we’ll move on to learning how to use Python cURL.
In this section, we’ll explore the basic cURL commands in Python. We will be focusing on making GET and POST requests. You’ll also learn how to perform these requests using the PycURL and Requests libraries. Additionally, we’ll also cover how to handle responses effectively and manage errors that might arise during HTTP requests.
a. Basic Commands with Requests:
1 2 3 4 5 6 7 |
# Basic Commands with Requests import requests get_response = requests.get('https://sampleurl.com') print(get_response.text) |
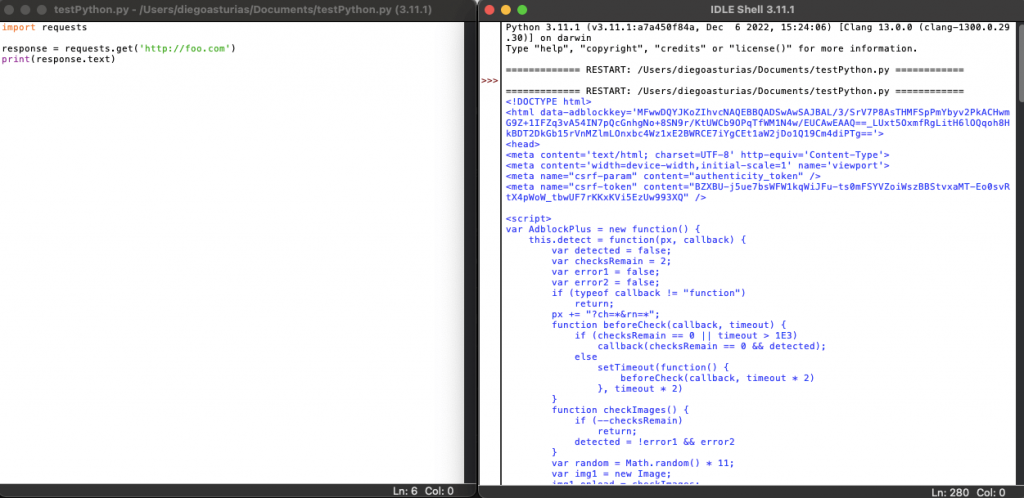
POST Request with Requests:
1 2 3 4 5 6 7 8 |
# POST Request with Requests import requests payload = {'param': 'value'} post_response = requests.post('https://sampleurl.com', data=payload) print(post_response.text) |
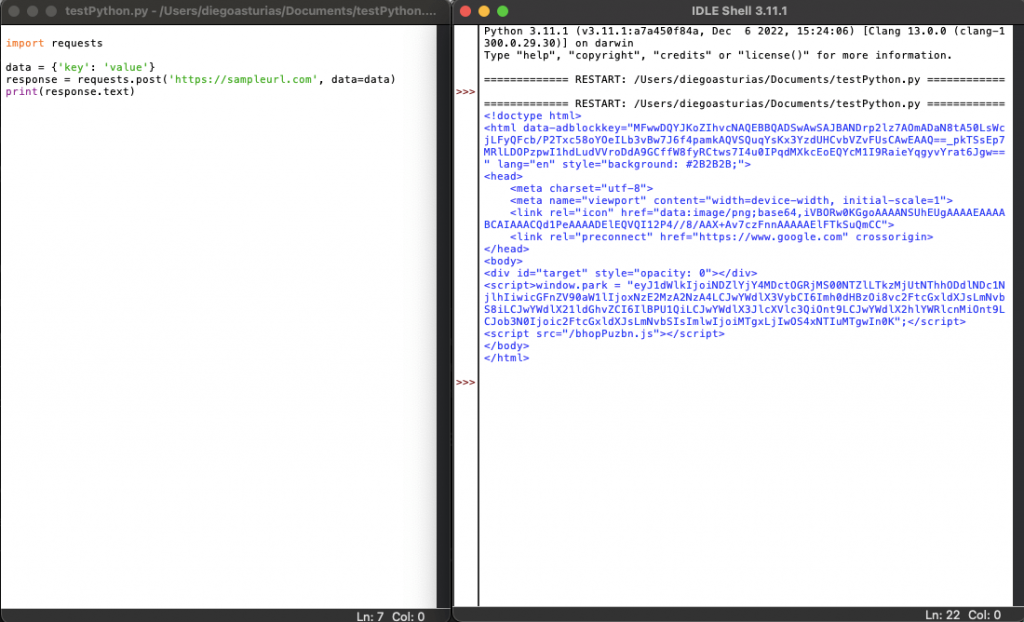
Handling Errors with Requests:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
import requests try: get_response = requests.get('https://example.com') get_response.raise_for_status() print(get_response.text) except requests.exceptions.HTTPError as http_err: print(f'HTTP error occurred: {http_err}') except Exception as err: print(f'Other error occurred: {err}') |
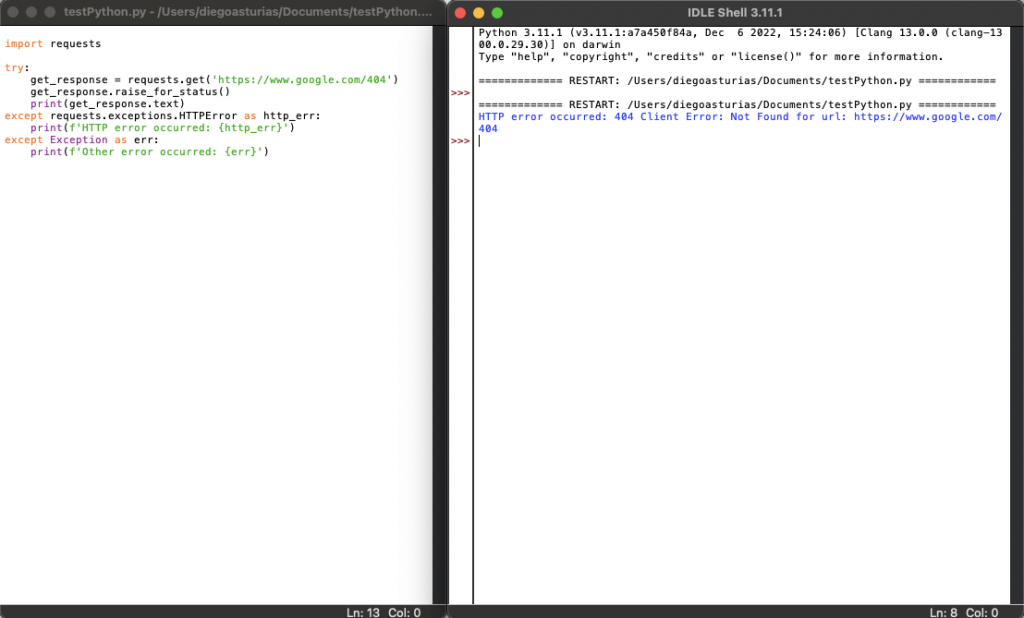
b. Basic Commands with PycURL.
Let’s say you already have PycURL installed. In this example setup, we will import the Curl class from the PycURL module and the BytesIO from the io module.
Note: The Curl class is crucial for making HTTP requests in Python using the PycURL library. It provides methods and attributes to set various options for the HTTP request, such as the URL, headers, and data. The BytesIO from the io module, on the other hand, is used to create an in-memory buffer. This buffer is like a placeholder that captures the response data from the HTTP request made by PycURL.
GET Request with PyURL
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
# GET Request with PyURL from pycurl import Curl from io import BytesIO curl_obj = Curl() response_buffer = BytesIO() curl_obj.setopt(curl_obj.URL, 'https://sampleurl.com') curl_obj.setopt(curl_obj.WRITEDATA, response_buffer) curl_obj.perform() curl_obj.close() response_body = response_buffer.getvalue().decode('utf-8') print(response_body) |
POST Request with PyURL.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
# POST Request with PycURL from pycurl import Curl from io import BytesIO from urllib.parse import urlencode curl_obj = Curl() response_buffer = BytesIO() payload = {'param': 'value'} encoded_payload = urlencode(payload) curl_obj.setopt(curl_obj.URL, 'https://sampleurl.com') curl_obj.setopt(curl_obj.POSTFIELDS, encoded_payload) curl_obj.setopt(curl_obj.WRITEDATA, response_buffer) curl_obj.perform() curl_obj.close() response_body = response_buffer.getvalue().decode('utf-8') print(response_body) |
Handling Responses with PycURL:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
# Handling Responses with PycURL from pycurl import Curl from io import BytesIO curl_obj = Curl() response_buffer = BytesIO() curl_obj.setopt(curl_obj.URL, 'https://sampleurl.com') curl_obj.setopt(curl_obj.WRITEDATA, response_buffer) curl_obj.perform() http_code = curl_obj.getinfo(curl_obj.RESPONSE_CODE) curl_obj.close() if http_code == 200: print("Success:", response_buffer.getvalue().decode('utf-8')) else: print("Error:", http_code) |
Need to “curl” up with some top-notch proxies for your Python projects?
Rapidseedbox offers high-success rate IPv4 and IPv6 proxies for fast, stable, and anonymous browsing. Benefit from high-end servers, dedicated bandwidth, and two proxy types (HTTPs & SOCKS5) ready for immediate use.
———
4. Advanced cURL Techniques
In this section, we’ll delve into advanced cURL techniques using Python. We’ll explore how to work with JSON data, customize HTTP headers, and handle file uploads and downloads. These techniques are crucial for more complex interactions with web APIs and services. They allow you to create more powerful and flexible scripts.
By mastering these advanced techniques, you will handle a wider range of tasks. For example, you’ll be able to optimize your web scraping and data interaction capabilities.
Advanced cURL Techniques with the Requests Library
a. Sending JSON Data with Requests:
1 2 3 4 5 |
import requests payload = {'param': 'value'} post_response = requests.post('https://sampleurl.com', json=payload) print(post_response.text) |
b. Custom Headers with Requests:
1 2 3 4 5 6 7 8 |
import requests headers = { 'User-Agent': 'CustomUserAgent', 'Accept': 'application/json' } get_response = requests.get('https://sampleurl.com', headers=headers) print(get_response.text) |
c. File Upload with Requests:
1 2 3 4 5 |
import requests files = {'file': open('path/to/your/file.txt', 'rb')} post_response = requests.post('https://sampleurl.com/upload', files=files) print(post_response.text) |
d. File Download with Requests:
1 2 3 4 5 |
import requests get_response = requests.get('https://sampleurl.com/file.txt') with open('downloaded_file.txt', 'wb') as f: f.write(get_response.content) |
Advanced cURL Techniques with the PycURL Library
a. Sending JSON Data with PycURL:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
from pycurl import Curl from io import BytesIO import json curl_obj = Curl() response_buffer = BytesIO() payload = {'param': 'value'} postfields = json.dumps(payload) curl_obj.setopt(curl_obj.URL, 'https://sampleurl.com') curl_obj.setopt(curl_obj.POSTFIELDS, postfields) curl_obj.setopt(curl_obj.HTTPHEADER, ['Content-Type: application/json']) curl_obj.setopt(curl_obj.WRITEDATA, response_buffer) curl_obj.perform() curl_obj.close() response_body = response_buffer.getvalue().decode('utf-8') print(response_body) |
b. Custom Headers with PycURL:
1 2 3 4 5 6 7 8 9 10 11 12 |
from pycurl import Curl from io import BytesIO curl_obj = Curl() response_buffer = BytesIO() curl_obj.setopt(curl_obj.URL, 'https://sampleurl.com') curl_obj.setopt(curl_obj.HTTPHEADER, ['User-Agent: CustomUserAgent', 'Accept: application/json']) curl_obj.setopt(curl_obj.WRITEDATA, response_buffer) curl_obj.perform() curl_obj.close() response_body = response_buffer.getvalue().decode('utf-8') print(response_body) |
c. File Upload with PycURL:
1 2 3 4 5 6 7 |
from pycurl import Curl curl_obj = Curl() curl_obj.setopt(curl_obj.URL, 'https://sampleurl.com/upload') curl_obj.setopt(curl_obj.HTTPPOST, [('file', (curl_obj.FORM_FILE, 'path/to/your/file.txt'))]) curl_obj.perform() curl_obj.close() |
d. File Download with PycURL:
1 2 3 4 5 6 7 8 9 10 11 12 |
from pycurl import Curl from io import BytesIO curl_obj = Curl() response_buffer = BytesIO() curl_obj.setopt(curl_obj.URL, 'https://sampleurl.com/file.txt') curl_obj.setopt(curl_obj.WRITEDATA, response_buffer) curl_obj.perform() curl_obj.close() with open('downloaded_file.txt', 'wb') as f: f.write(response_buffer.getvalue()) |
5. Best Practices, Tips, and Troubleshooting
The following is a compiled list of the best practices and troubleshooting steps. You can use these to handle and resolve common issues when using Python cURL. Take these tips to ensure secure, efficient, and reliable HTTP requests in your applications.
a. Security Considerations.
- Use HTTPS: Always use HTTPS (HTTP over SSL/TLS) to ensure data encryption during transmission.
- Validate SSL Certificates: Ensure SSL certificates are valid to prevent man-in-the-middle attacks.
- Handle Sensitive Data Securely: Avoid hardcoding sensitive information like API keys and credentials. Instead, use environment variables.
b. Optimizing Performance.
- Persistent Connections: Reuse connections to reduce overhead. Use the sessions in the Requests library.
- Timeout Settings: Always set appropriate timeouts to prevent hanging requests.
- Limit Redirects: Control the number of redirects to avoid unnecessary network usage.
- Minimize Data Transfer: Use query parameters and request only the necessary data fields.
c. Debugging Tips:
- Verbose Output: Enable verbose output in cURL to see detailed request and response information.
- Check Response Codes: Always check HTTP response codes. This way you can understand the request’s outcome.
- Log Requests: Keep the logs of requests and responses close by. You’ll use them for later analysis.
d. Common Errors and Their Solutions:
- SSL Certificate Errors:
- Error: SSL certificate problem. (You are unable to get a local issuer certificate.)
- Solution: Ensure that the correct CA bundle is installed. Use certifi in Python to handle SSL certificates.
- Timeout Errors:
- Error: Request timed out.
- Solution: Increase timeout settings. Use the timeout parameter in Requests or TIMEOUT option in PycURL.
- Authentication Errors:
- Error: 401 Unauthorized.
- Solution: Check your authentication credentials and ensure they are ok. Also, we recommend using proper headers for token-based authentication.
- Connection Errors:
- Error: Failed to connect to host.
- Solution: Verify the server URL and network connectivity. Ensure that the server is up and running.
- Invalid URL Errors:
- Error: URL using bad/illegal format or missing URL.
- Solution: Ensure the URL is correctly formatted and encoded.
6. FAQ for Python cURL
a. What are some real-world examples of using Python cURL?
Python cURL can be used for web scraping, testing APIs, downloading data files, and automating data transfer tasks.
b. How can I perform web scraping with cURL in Python?
You can use PycURL or the Requests library to fetch web page content and parse it with libraries like BeautifulSoup. Check the following guide to learn more about BeautifulSoup and Web Scraping.
c. How do I use cURL with APIs in Python?
You can make API requests using PycURL or Requests. With the Requests library, you can easily handle HTTP methods such as GET and POST with simple, readable code. For more advanced use cases requiring detailed control use the PycURL. The former provides robust options to manage requests and responses efficiently.
d. How do I make API requests with cURL in Python?
Use the Requests library for easier syntax or PycURL for more control. Set the URL, HTTP method, headers, and body as needed.
e. How do I handle authentication and headers with cURL in Python?
For handling the authentication, use basic, token-based, or OAuth methods. For customizing headers, include content types, user agents, and authorization tokens.
f. What are the common HTTP methods used in Python cURL?
GET, POST, PUT, DELETE, and PATCH are common with Python cURL for interacting with APIs.
g. What tools can I use for web scraping with Python cURL?
We recommend BeautifulSoup, Scrapy, and Selenium. These three are popular tools for parsing and extracting data from web pages.
h. How do I handle data extraction using cURL in Python?
Fetch the data using cURL, parse the response, and then extract the required information using libraries like BeautifulSoup. Learn more about this process in the Ultimate Guide to Web Scraping.
i. How do I customize HTTP headers in Python cURL?
Set custom headers using the setopt method in PycURL or the headers parameter in Requests.
j. What is the role of REST API in Python cURL?
REST APIs allow you to interact with web services using standard HTTP methods, making cURL a powerful tool for API consumption.
7. Final Words.
Python cURL makes data transfer and web API interactions so easy thanks to libraries like Requests and PycURL. These libraries have you covered if you are looking to improve your web scraping, data processing, and more.
This guide provided a thorough overview of using cURL within Python for various tasks. It also highlights setting up cURL with PycURL and Requests, executing basic and advanced commands, and handling responses and errors. In addition, by following the best practices and troubleshooting tips outlined in this guide, you can ensure efficient, secure, and reliable HTTP requests in your Python applications.
We hope you found this guide informative and useful. If you have any suggestions or questions about this article, please leave them in the comments section below.
0Comments